How to protect an Angular standalone component application against bot attacks with CaptchaFox
Last updated on November 23, 2023
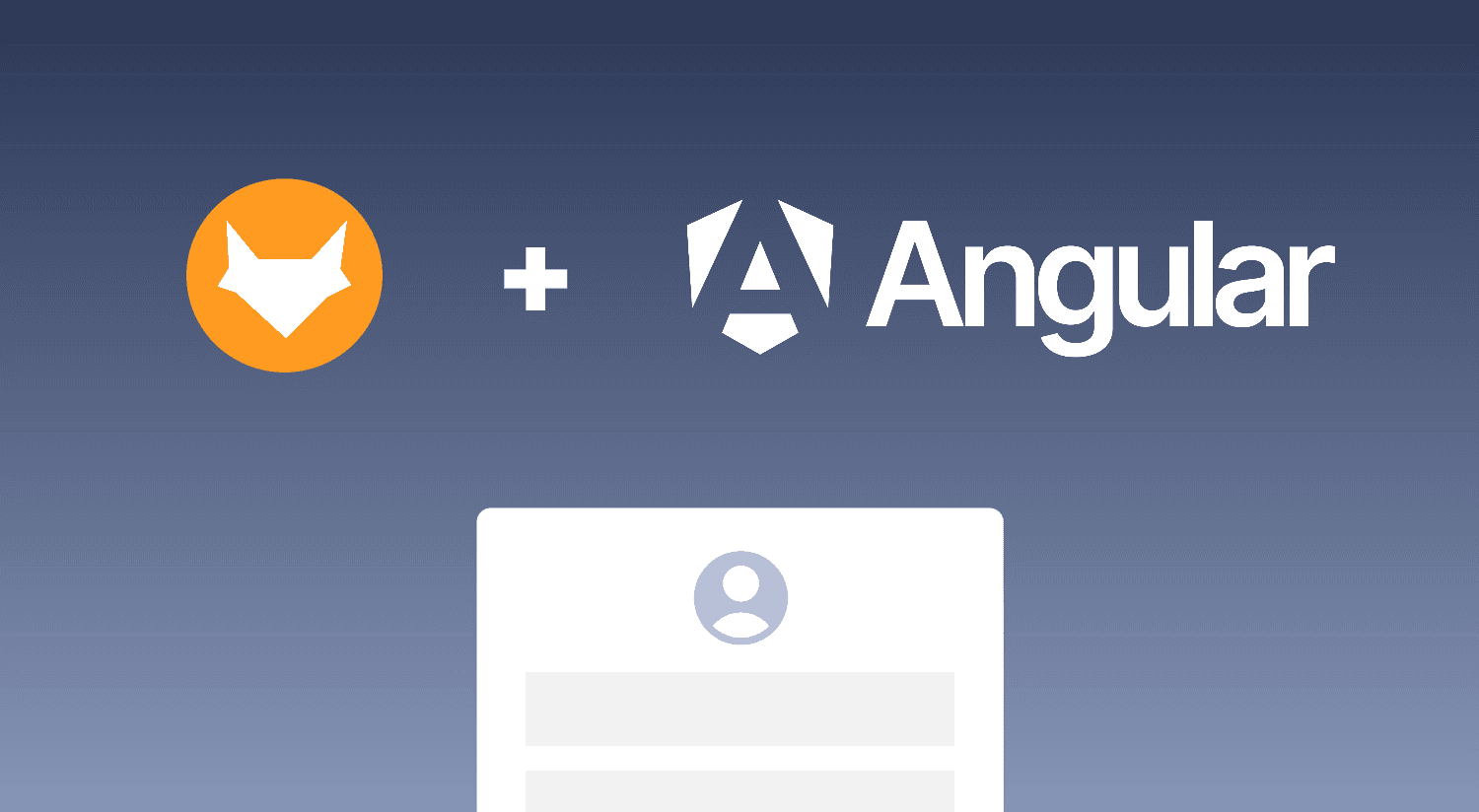
In this article, we explain how to integrate the CaptchaFox widget into an Angular standalone component. With our provided library, you can make your application more secure by asking users to perform a CAPTCHA check before submitting forms.
What is Angular?
Angular is a Typescript-based framework for the development of scalable web applications. The focus of Angular is on architecture, which is why it comes with numerous libraries for routing or client-server communication, for example.
What are standalone components
Standalone components do not require NGModules for use. This has the advantage that they can be used in the entire application without having to declare them in modules. This saves unnecessary code and administrative effort.
Getting started
Step 1: Installing CaptchaFox
To get started, you need to install the CaptchaFox package with your favourite package manager. Open your terminal in your project and execute the following command:
npm install @captchafox/angular
Step 2: Enable CaptchaFox in the app
To use the component in the entire Angular project, you must now add the component to appConfig.ts
and add the site key you have generated to forRoot()
. You can also use our test key for tests on e.g. localhost.
import { ApplicationConfig, importProvidersFrom } from '@angular/core';
import { provideRouter } from '@angular/router';
import { CaptchaFoxModule } from '@captchafox/angular';
import { routes } from './app.routes';
export const appConfig: ApplicationConfig = {
providers: [provideRouter(routes),
importProvidersFrom(CaptchaFoxModule.forRoot({
siteKey: '<YOUR_SITE_KEY>'
}))
]
};
Step 3: Creating the form
Next, create a form in your Angular application. You can do this by generating a new component and adding the form elements. To be able to use these, we first add the FormBuilder in the constructor. Then we create the form group.
import { Component } from '@angular/core';
import { CommonModule } from '@angular/common';
import { FormBuilder, ReactiveFormsModule } from '@angular/forms';
@Component({
selector: 'app-form',
standalone: true,
imports: [CommonModule, ReactiveFormsModule],
templateUrl: './form.component.html',
styleUrl: './form.component.scss'
})
export class FormComponent {
constructor(private formBuilder: FormBuilder) {}
registerForm = this.formBuilder.group({
mail: '',
password: '',
})
}
Now the form can also be added to the corresponding HTML:
<form [formGroup]="registerForm" (ngSubmit)="onSubmit()">
<div>
<label for="mail">
E-Mail
</label>
<input id="mail" type="mail" formControlName="mail">
</div>
<div>
<label for="password">
Password
</label>
<input id="password" type="password" formControlName="password">
</div>
<button class="button" type="submit">Register</button>
</form>
We have now added two input fields and a button. Next, we will create an onSubmit method for the latter. In the example, this method will only empty the form and not submit it.
onSubmit() {
this.registerForm.reset();
// Your business logic
}
Step 4: Add CaptchaFox to the form
As you have configured the component for the entire project in step 2, you only need to import the module into your component, which then looks like this:
@Component({
selector: 'app-form',
standalone: true,
imports: [CommonModule, ReactiveFormsModule, CaptchaFoxModule],
templateUrl: './form.component.html',
styleUrls: ['./form.component.css']
})
Now you can also integrate the CatpchaFox component into your form. To do this, simply use the HTML tag <ngx-captchafox>
and add the field name using the formControlName
attribute.
<form [formGroup]="registerForm" (ngSubmit)="onSubmit()">
<div>
<label for="mail">
E-Mail
</label>
<input id="mail" type="mail" formControlName="mail">
</div>
<div>
<label for="password">
Password
</label>
<input id="password" type="password" formControlName="password">
</div>
<ngx-captchafox formControlName="captchaToken"></ngx-captchafox>
<button class="button" type="submit">Register</button>
</form>
Finally, you must add the field to your form group. This could then look like this:
@Component({
selector: 'app-form',
standalone: true,
imports: [CommonModule, ReactiveFormsModule, CaptchaFoxModule],
templateUrl: './form.component.html',
styleUrl: './form.component.scss'
})
export class FormComponent {
constructor(private formBuilder: FormBuilder) {}
registerForm = this.formBuilder.group({
mail: '',
password: '',
captchaToken: new FormControl(null)
})
onSubmit() {
this.registerForm.reset();
// Your business logic
}
}
Make sure to validate the received token on your backend before letting the user perform the action. You can read more about the site verification API in the documentation.
Next steps
You have now successfully integrated CaptchaFox into your Angular standalone component!
You can customise the code according to your requirements and protect additional Formluare in your Angular application.
If you want to dive deeper into the integration, you can read the official documentation.