How to protect a Solid.js application against bot attacks with CaptchaFox
Last updated on February 6, 2024
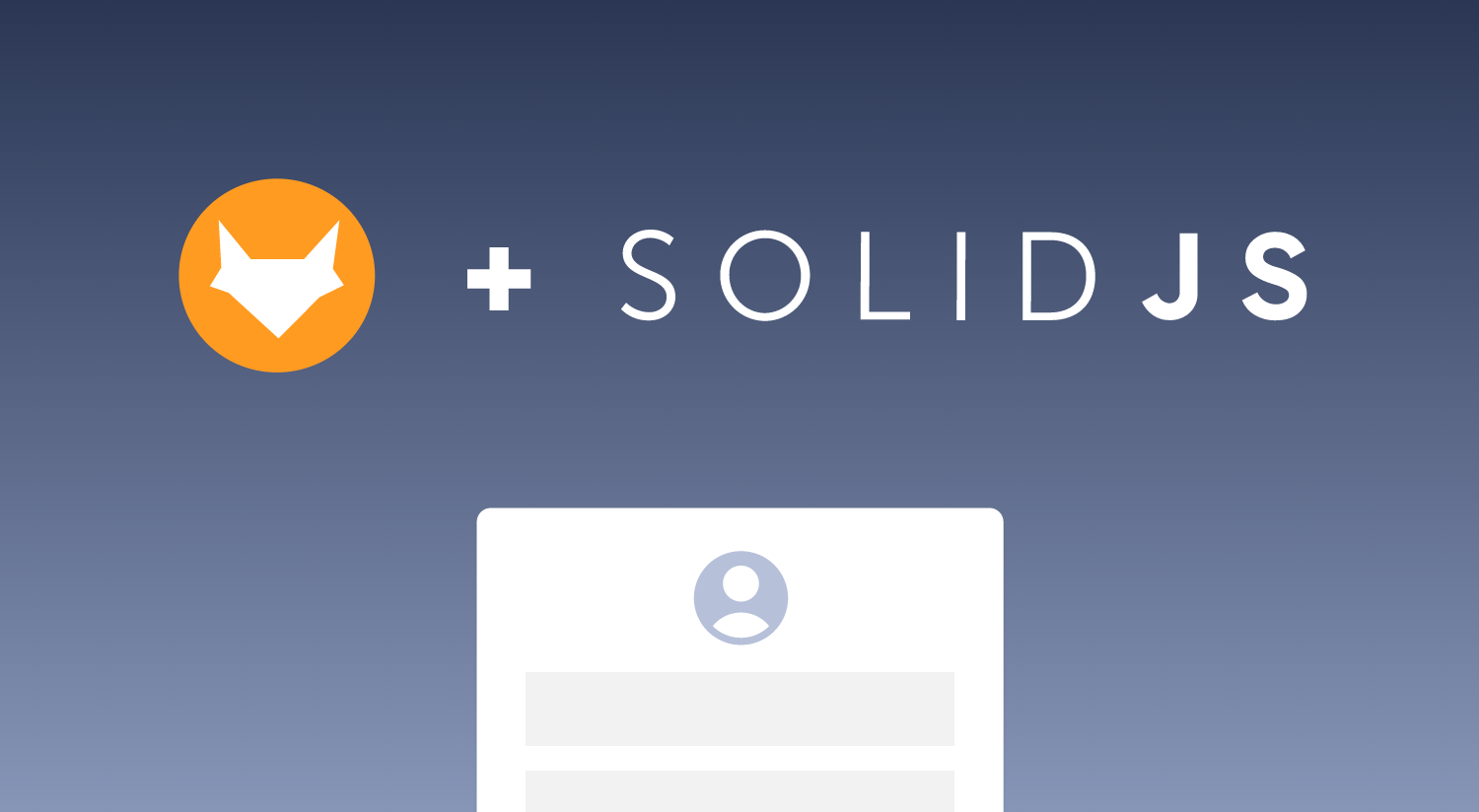
In this article, we explain how to integrate the CaptchaFox widget into a Solid.js application. With our provided library, you can make your application more secure by asking users to perform a CAPTCHA check before submitting forms.
What is Solid?
Solid is a fairly new JavaScript framework for creating web applications. Just like React, it uses JSX and provides similar APIs. It is characterised above all by very good browser performance and a simple structure. The framework relies heavily on fine-grained reactivity, which means that a virtual DOM is not necessary.
Step 1: Installation of CaptchaFox
To get started, you need to install the CaptchaFox package with your preferred package manager. Open your terminal in your project and execute the following command:
npm install @captchafox/solid
Step 2: Creating a form
Next, create a form in your application. You can do this by creating a new component and adding the form elements. Here is an example of a simple form:
function ContactForm() {
return (
<form>
<label htmlFor="name">Name:</label>
<input type="text" id="name" name="name" />
<label htmlFor="email">Email:</label>
<input type="email" id="email" name="email" />
<button type="submit">Submit</button>
</form>
)
}
This form contains two input fields for the user's name and e-mail address as well as a button for submitting.
Step 3: Add CaptchaFox to the form
Now that you have a form, the CaptchaFox widget can be added. To do this, import the component from the @captchafox/solid
package and add it to your form. The following code is an example of what the form will look like afterwards. The page key must be replaced with your key from the admin portal.
import { CaptchaFox } from '@captchafox/solid'
function ContactForm() {
return (
<form>
<label htmlFor="name">Name:</label>
<input type="text" id="name" name="name" />
<label htmlFor="email">Email:</label>
<input type="email" id="email" name="email" />
<CaptchaFox sitekey="YOUR_SITEKEY" />
<button type="submit">Submit</button>
</form>
)
}
Step 4: Verification
You can then add a method to process the result of the captcha and to check if the verification was successful.
import { CaptchaFox } from '@captchafox/solid'
function ContactForm() {
const handleVerify = (token: string) => {
// Your business logic
}
return (
<form>
<label htmlFor="name">Name:</label>
<input type="text" id="name" name="name" />
<label htmlFor="email">Email:</label>
<input type="email" id="email" name="email" />
<CaptchaFox sitekey="YOUR_SITEKEY" onVerify={handleVerify}/>
<button type="submit">Submit</button>
</form>
)
}
For verification, the received token must be validated in your backend before you let the user perform the action. You can find more information about the API for validation in the getting started guide.
Next steps
You have now successfully integrated CaptchaFox into your Solid.js application!
You can customize the code according to your requirements and protect additional forms in your application.
If you want to dive deeper into the integration, you can read the official documentation.