How to protect a Next.js application against bot attacks with CaptchaFox
Last updated on October 23, 2023
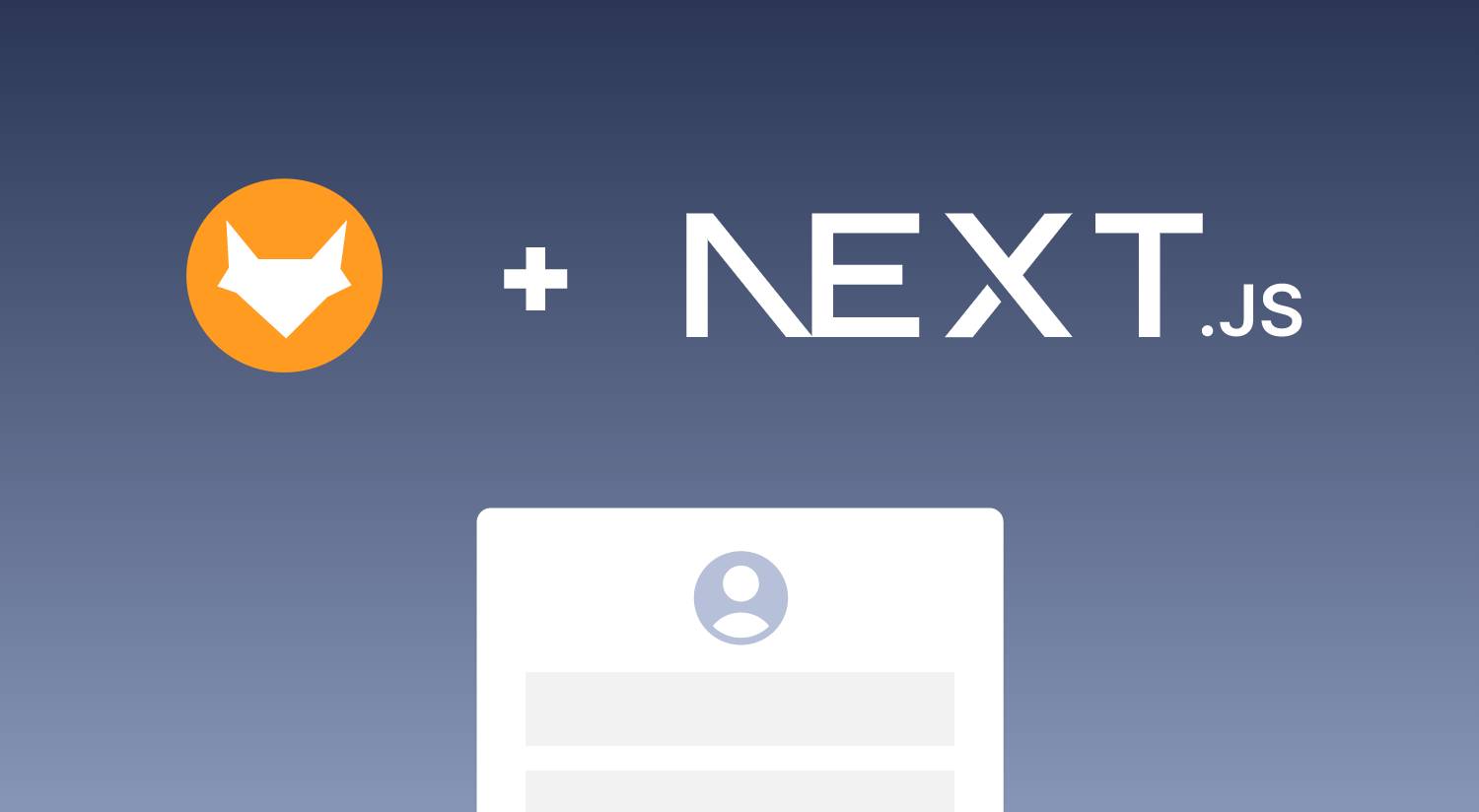
In this post, we will guide you through the process of integrating the CaptchaFox widget into a Next.js application using our pre-built library. This will help you add a layer of security to your application by requiring users to complete a CAPTCHA challenge before submitting a form.
What is Next.js?
Next.js is a popular React-based framework for building server-rendered, statically generated, and performance-optimized web applications. It provides a set of features and tools that make it easier to build universal (client-side and server-side rendered) applications with React.
Next.js applications are built using a combination of React components and server-side rendering, which allows for improved SEO and performance. The framework also includes automatic code splitting, optimized static deployment, and support for a wide range of third-party libraries and integrations. You can read more about Next.js on their official website.
Getting started
Step 1: Installing CaptchaFox
To get started, you'll need to install the CaptchaFox package using your preferred package manager. Open your terminal inside your project and run the following command:
npm install @captchafox/react
Step 2: Creating a Form
Next, create a form in your Next.js application. You can do this by creating a new component and adding the form elements to it. Here's an example of a simple form:
function ContactForm() {
return (
<form>
<label htmlFor="name">Name:</label>
<input type="text" id="name" name="name" />
<label htmlFor="email">Email:</label>
<input type="email" id="email" name="email" />
<button type="submit">Submit</button>
</form>
)
}
This form includes two input fields for the user's name and email, and a submit button.
Step 3: Adding CaptchaFox to the Form
Now that you have a form, you can add the CaptchaFox component to it. You can do this by importing the component from the @captchafox/react
package and adding it to your form component. Here's an example of how to do this:
import { CaptchaFox } from '@captchafox/react'
function ContactForm() {
return (
<form>
<label htmlFor="name">Name:</label>
<input type="text" id="name" name="name" />
<label htmlFor="email">Email:</label>
<input type="email" id="email" name="email" />
<CaptchaFox sitekey="YOUR_SITEKEY" />
<button type="submit">Submit</button>
</form>
)
}
Here we've added the CaptchaFox component to the form and given it a sitekey prop. Replace YOUR_SITEKEY
with the sitekey you received after creating the site in the CaptchaFox Portal. This key identifies your site and enables the CAPTCHA challenge.
Step 4: Validating the request
After the challenge has been solved, a special key is added to the form data which contains the response token. You need to verify this token on your server to ensure that the request is legitimate.
In Next.js, you can use API routes to do this.
First, create a new API route in the api
folder, e.g. /api/contact.ts
:
import type { NextApiRequest, NextApiResponse } from 'next';
export default async function handler(req: NextApiRequest, res: NextApiResponse) {
const body = req.body;
if (!body.name || !body.email) {
return res.status(400).json({ error: 'Missing request properties' });
}
res.redirect('/thanks');
}
We also need to update our form component so that it sends the response to our API route. Add the following attributes to the form
element:
<form method="POST" action="/api/contact">
Then call the CaptchaFox siteverify
endpoint and pass it the response token and your organisation secret key. To simplify this process, we'll use the @captchafox/node
package and its verify
function:
import type { NextApiRequest, NextApiResponse } from 'next';
import { CAPTCHA_RESPONSE_KEY, verify } from '@captchafox/node';
// You should store your secret in an env variable
const CAPTCHAFOX_SECRET = 'ORG_SECRET_KEY';
export default async function handler(req: NextApiRequest, res: NextApiResponse) {
const body: Data = req.body;
if (!body.name || !body.email) {
return res.status(400).json({ error: 'Missing request properties' });
}
const responseToken = body[CAPTCHA_RESPONSE_KEY];
// return bad request if token is missing
if (!responseToken) {
return res.status(400).json({ error: 'Captcha Token missing' });
}
// send request to verify token
const verifyResponse = await verify(CAPTCHAFOX_SECRET, responseToken);
// Not sucessful -> Send error response
if (!verifyResponse.success) {
const errorCodes = verifyResponse['error-codes'];
return res.status(401).json({ error: 'Invalid captcha response', codes: errorCodes });
}
res.redirect('/thanks');
}
This api route will now check if the response token exists and is valid. If successful, it redirects the user to a "thank you" page. If there is an error, it returns it as a json response.
Next steps
You have now successfully protected a form in your Next.js application against bot attacks using CaptchaFox! Be sure to customise the code to fit your business needs and secure more forms in your Next.js application.
If you want to use CaptchaFox with a popular form library like react-hook-form, you can check out our detailed example in the Javascript Integrations repository.
If you want to dive deeper into the integration, you can read the official documentation.
About CaptchaFox
CaptchaFox is a GDPR-compliant solution based in Germany that protects websites and applications from automated abuse, such as bots and spam. Its distinctive, multi-layered approach utilises risk signals and cryptographic challenges to facilitate a robust verification process. CaptchaFox enables customers to be onboarded in a matter of minutes, requires no ongoing management and provides enterprises with long-lasting protection.
To learn more about CaptchaFox, talk to us or start integrating our solution with a free trial.